Hi Stephen,
Currently, the Zernike analyses do not have a Data Series or Data Grid available to them. There are a few ways to confirm this, but my favorite way is to investigate the newZern_Results object with a breakpoint. I've shown what that looks like in the Forum post 'How to extract the result of ImageSimulation in ZOS-API.' When we investigate it, we see that each analysis data type has a '0' next to it. This means that there is no data to retrieve:
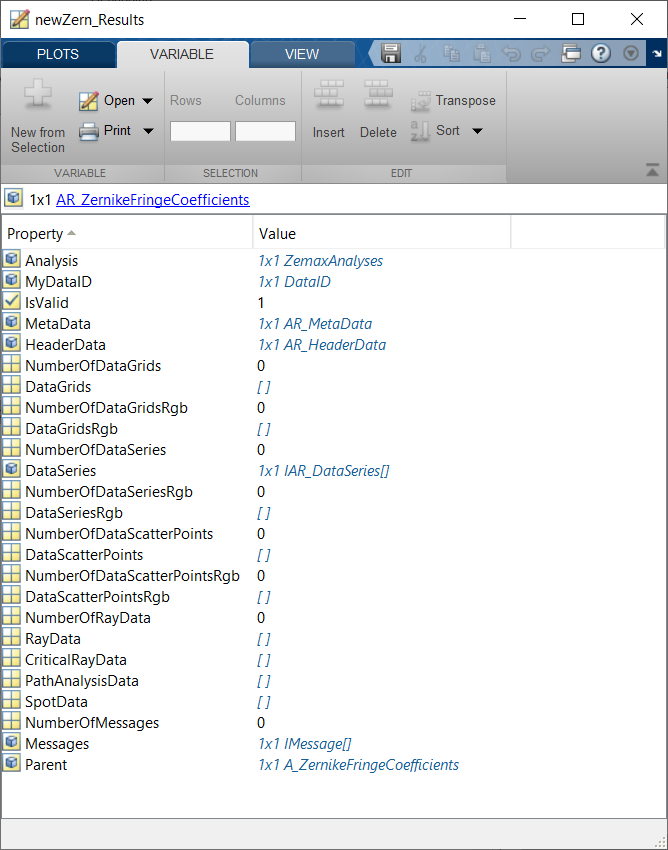
(Note: each analysis will have a results interface like this. To quickly determine which data sets are available to a particular analysis, check out the Knowledgebase article 'Generating a list of output data types for each analysis in the ZOS-API.')
At this point, you have two options. The most straightforward is to extract the data by using the property GetTextFile. This command will generate a text file with a user-defined filename, which will match the output you see under the 'Text' tab of the Zernike Fringe Coefficients analysis window. Once the text file is generated, you can use Matlab to parse it for the coefficient values.
Alternatively, you could use the ZERN optimization operand. This operand will be able to pull the same values you see in the Zernike Fringe Coefficients tool. I previously wrote an example of this procedure for the Zernike Standard Coefficients. You can find it below:
TheSystem = TheApplication.PrimarySystem;
TheMFE = TheSystem.MFE
% Declare operand settings
term = 4;
pwave = 0;
for a = 1:TheSystem.SystemData.Wavelengths.NumberOfWavelengths
if TheSystem.SystemData.Wavelengths.GetWavelength(a).IsPrimary
pwave = a;
end
end
sample = 2;
field = 1;
% Choose the Zernike type we're lookiing at
% 0 = Fringe, 1 = Standard, 2 = Annular
type = 1;
epsilon = 0;
vertex = 0;
% Get operand value
testVal = TheMFE.GetOperandValue(ZOSAPI.Editors.MFE.MeritOperandType.ZERN, term, pwave, sample, field, type, epsilon, vertex, 0);
Using the function GetOperandValue, we can extract any optimization operand value without including it in the Merit Function. In the above code, I am pulling the 4th Zernike Standard Coefficient term into Matlab. You’ll see by the variables I’ve declared that this operand asks for the term of interest, wavelength, sampling rate, field, and type:
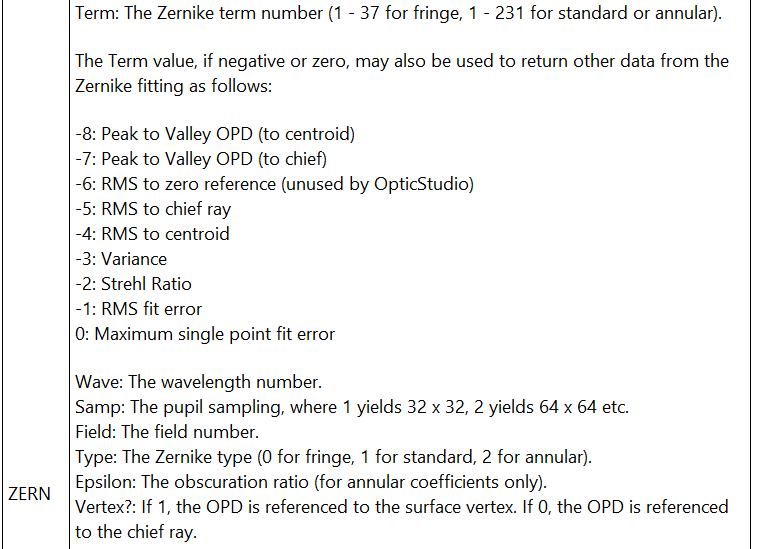
Using the above, you could write a loop to cycle through the Zernike terms and store them as an array in Matlab. This method will pull the same terms you see within the Zernike coefficient analysis you’re using (assuming all settings are the same). This will take a little longer than simply pulling the text file of data, but will leave you with a Matlab-native object of double-precision values.
Let me know if you have any questions about this!
Best,
Allie
PS: That's a great icon you have. Corgis are the best!