When dealing with non-Standard surface types, you typically have access to extra parameters that are specific to that surface. For example, the Coordinate Break surface provides inputs for Decenter X, Decenter Y, etc.

To access these parameters in the API, our sample code offers two options:
- Access a parameter based on the parameter number. A typical command for this may look like the following: decenterX = TheSystem.LDE.GetSurfaceAt(1).GetSurfaceCell(ZOSAPI.Editors.LDE.SurfaceColumn.Par1).DoubleValue;
- Access a parameter based on its column number. A typical command for this may look like the following: decenterX = TheSystem.LDE.GetSurfaceAt(1).GetCellAt(12).DoubleValue;
In both of these cases, you will need to have prior knowledge of the location in the LDE of the parameters you want to change. Or you will need to query the “Header” of the column you’re working with to confirm the parameter is correct.
There is a third surface-specific method for calling parameters by their title. This method utilizes the ISurface interface within the LDE namespace. The steps for accessing this are below:
- Access a surface with the GetSurfaceAt property
- Access the ISurface interface with the SurfaceData command
- If needed, cast to the ISurface interface with the interface of the surface type (required for C#, not required for Matlab or PythonNET)
- Call a parameter from this interface. This allows you to call with the name “Decenter_X” instead of with a column or parameter number.
The commands for surface-specific parameters are found in the corresponding ISurfaceXXX interface within the LDE Namespace:
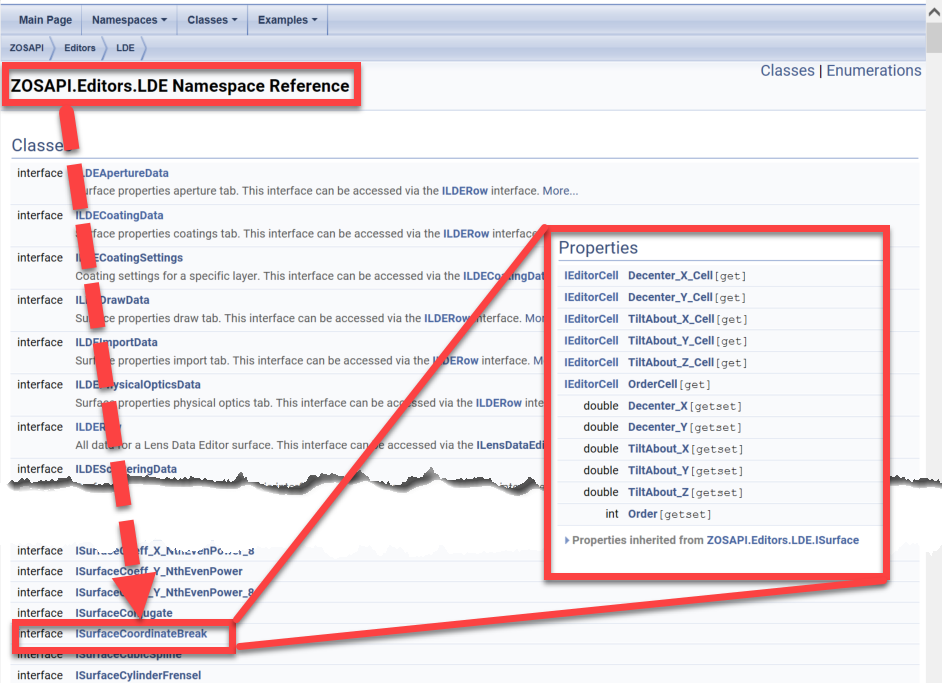
Below, I am providing examples in some of our supported languages.
Python .NET
Hide contentShow content
surf1 = TheSystem.LDE.GetSurfaceAt(1)
surfData = surf1.SurfaceData
surfData.Decenter_X = 10
Matlab
Hide contentShow content
surf1 = TheSystem.LDE.GetSurfaceAt(1);
surfData = surf1.SurfaceData;
surfData.Decenter_X = 20;
C#
Hide contentShow content
using System;
using ZOSAPI;
using ZOSAPI.Editors;
using ZOSAPI.Editors.LDE;
…
ILensDataEditor TheLDE = TheSystem.LDE;
ILDERow surf1 = TheLDE.GetSurfaceAt(1);
ISurface surf1Data = surf1.SurfaceData;
// We must cast to the specific surface interface from the ISurface interface
var coordBreakParams = (ISurfaceCoordinateBreak)surf1Data;
coordBreakParams.Decenter_X = 30;
double decenterXVal = coordBreakParams.Decenter_X;
Console.WriteLine(decenterXVal);
Console.ReadKey();