I’m writing a user-defined merit function operand in C++ and would like to read the header lines from an analysis window (and then parse the results to extract numerical values of interest). For example, in POP, the header contains several useful numbers related to the analysis results.
Using Matlab, this is fairly simple as
However, it appears that in C++ the header lines are passed using SAFEARRAY. There is one example (#23) that shows how this is done for numerical arrays (see below).
Just curious if someone might know how to do something similar for the header lines? Is it possible to get the header lines in the form of a string array? If so, a simple example would be greatly appreciated!
Thanks,
Jeff
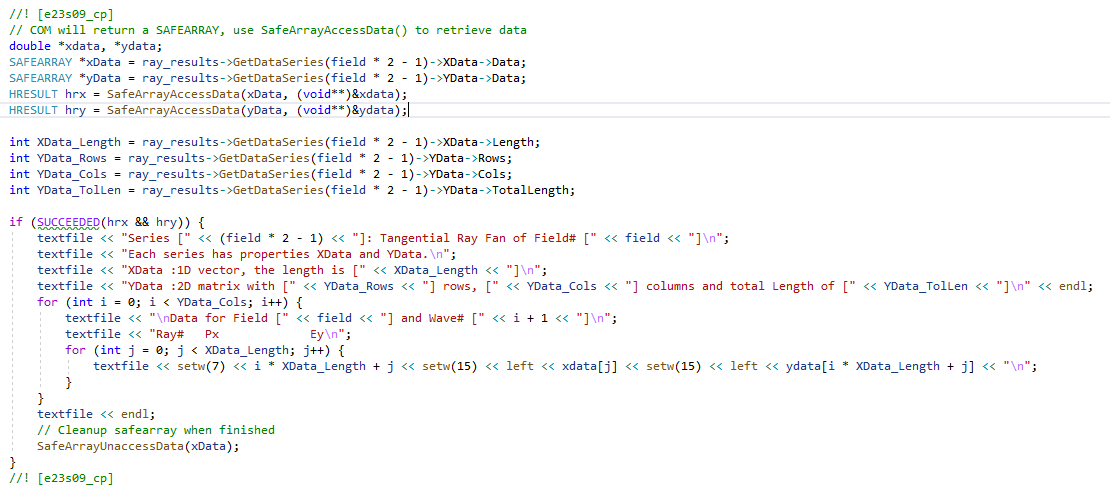
Best answer by MichaelH
View original